App Shortcuts
Shortcuts deliver specific types of content to your users by helping them quickly access parts of your app.1
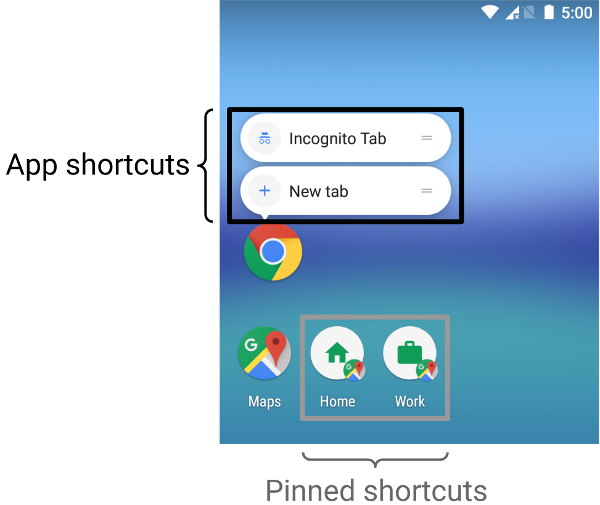
As a developer, you can define shortcuts to perform specific actions in your app. You can display these shortcuts in a supported launcher or assistant—like Google Assistant—and help your users quickly start common or recommended tasks within your app.2
Each shortcut references one or more intents, each of which launches a specific action in your app when users select the shortcut. The types of shortcuts you create for your app depend on the app’s use case.3
You can publish the following types of shortcuts for your app:4
- Static shortcuts are defined in a resource file that is packaged into an APK or app bundle;
- Dynamic shortcuts can be pushed, updated, and removed by your app only at runtime;
- Pinned shortcuts can be added to supported launchers at runtime if the user grants permission.
Static shortcuts
Static shortcuts are best for apps that link to content using a consistent structure throughout the lifetime of a user’s interaction with the app. Because most launchers only display four shortcuts at once, static shortcuts are useful for performing a routine task in a consistent way, such as if the user wants to view their calendar or email in a specific way.
To create a static shortcut, do the following:
- In your app’s
AndroidManifest.xml
file, find the activity whose intent filters are set to theandroid.intent.action.MAIN
action and theandroid.intent.category.LAUNCHER
category; - Add a
<meta-data>
element to this activity that references the resource file where the app’s shortcuts are defined; - Create a new resource file called
res/xml/shortcuts.xml
; - In the new resource file, add a
<shortcuts>
root element that contains a list of<shortcut>
elements. In each<shortcut>
element, include information about a static shortcut including its icon, description labels, and the intents it launches within the app.
Dynamic shortcuts
Dynamic shortcuts provide links to specific, context-sensitive actions within your app. These actions can change between uses of your app and while your app is running. Good uses for dynamic shortcuts include calling a specific person, navigating to a specific location, and loading a game from the user’s last save point. You can also use dynamic shortcuts to open a conversation.
The ShortcutManagerCompat
Jetpack library is a helper for the ShortcutManager
API, which lets you manage dynamic shortcuts in your app. Using the ShortcutManagerCompat library reduces boilerplate code and helps ensure that your shortcuts work consistently across Android versions.
The ShortcutManagerCompat
API lets your app perform the following operations with dynamic shortcuts:
- Push and update: use
pushDynamicShortcut()
to publish and update your dynamic shortcuts. If there are already dynamic or pinned shortcuts with the same ID, each mutable shortcut updates; - Remove: remove a set of dynamic shortcuts using
removeDynamicShortcuts()
. Remove all dynamic shortcuts usingremoveAllDynamicShortcuts()
.
Here’s an example of creating a dynamic shortcut and associating it with your app:
val shortcut = ShortcutInfoCompat.Builder(context, "id1")
.setShortLabel("Website")
.setLongLabel("Open the website")
.setIcon(IconCompat.createWithResource(context, R.drawable.icon_website))
.setIntent(Intent(Intent.ACTION_VIEW,
Uri.parse("https://www.mysite.example.com/")))
.build()
ShortcutManagerCompat.pushDynamicShortcut(context, shortcut)
Pinned shortcuts
On Android 8.0 (API level 26) and higher, you can create pinned shortcuts. Unlike static and dynamic shortcuts, pinned shortcuts appear in supported launchers as separate icons.
To pin a shortcut to a supported launcher using your app, complete the following steps:
- Use
isRequestPinShortcutSupported()
to verify that the device’s default launcher supports in-app pinning of shortcuts; - Create a
ShortcutInfo
object in one of two ways, depending on whether the shortcut exists:- If the shortcut exists, create a
ShortcutInfo
object that contains only the existing shortcut’s ID. The system finds and pins all other information related to the shortcut automatically; - If you’re pinning a new shortcut, create a
ShortcutInfo
object that contains an ID, an intent, and a short label for the new shortcut.
- If the shortcut exists, create a
- Pin the shortcut to the device’s launcher by calling
requestPinShortcut()
. During this process, you can pass in aPendingIntent
object, which notifies your app only when the shortcut pins successfully. After a shortcut is pinned, your app can update its contents using theupdateShortcuts()
method.
Best practices for shortcuts
- Follow the design guidelines. To make your app’s shortcuts visually consistent with the shortcuts used for system apps, follow the App Shortcuts Icon Design Guidelines;
- Publish only four distinct shortcuts. Although the API supports a combination of up to 15 static and dynamic shortcuts for your app, we recommend that you publish only four distinct shortcuts, to improve their visual appearance in the launcher;
- Limit shortcut description length. The space in the menu that shows your app’s shortcuts in the launcher is limited. When possible, limit the length of the “short description” of a shortcut to 10 characters and limit the length of the “long description” to 25 characters;
- Maintain shortcut and action usage history. For each shortcut you create, consider the different ways a user can accomplish the same task directly within your app. Call
reportShortcutUsed()
in each of these situations so that the launcher maintains an accurate history of how frequently a user performs the actions representing your shortcuts; - Update shortcuts only when their meaning is retained. When changing dynamic and pinned shortcuts, only call updateShortcuts() when changing the information of a shortcut that retains its meaning;
- Check dynamic shortcuts whenever you launch your app. Dynamic shortcuts aren’t preserved when the user restores their data onto a new device. For this reason, we recommend that you check the number of objects returned by
getDynamicShortcuts()
each time you launch your app and re-publish dynamic shortcuts as needed.
Links
Further reading
Extend dynamic shortcuts to Google Assistant with App Actions